But being in its first days (the beta has just been released!) a bit of documentation is still missing, and on the internet there aren’t many examples on how to write code.
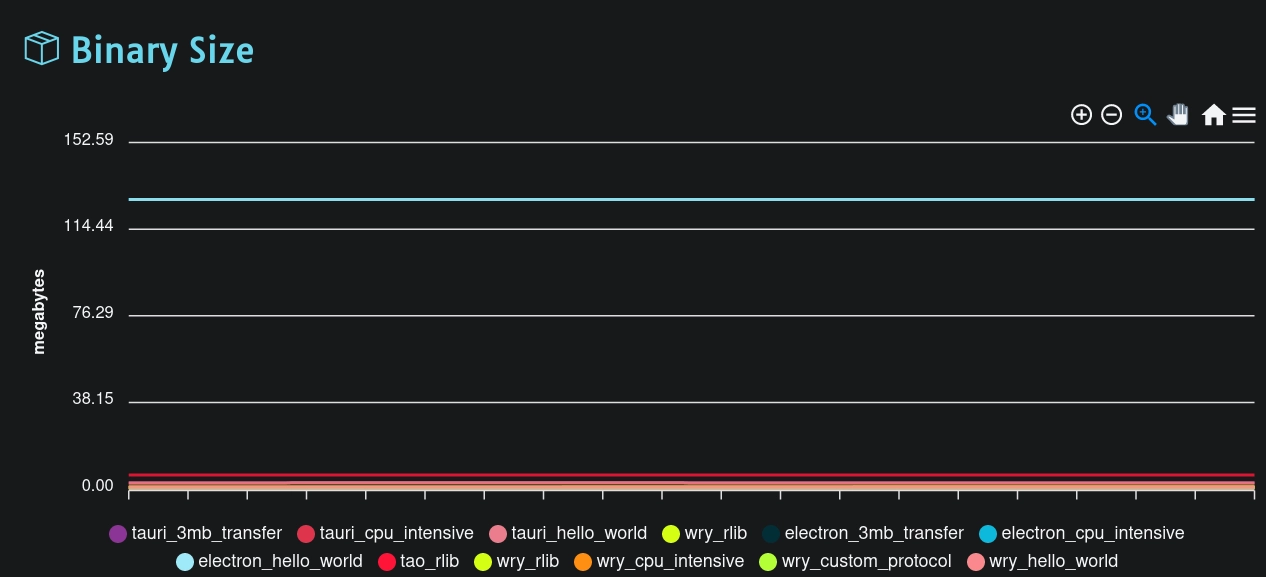
One thing that took me a bit of time to figure out, is how to read environment variables from the frontend of the application (JavaScript / Typescript or whichever framework you are using).
The Command
As usual, when you know the solution the problem seems easy. In this case, we just need an old trick: delegating!
In particular, we will use the Command
API to spawn a sub-process (printenv
) and reading the returned value. The code is quite straightforward, and it is synchronous:
1import { Command } from "@tauri-apps/api/shell"; 2 3async readEnvVariable(variableName: string): Promise<string> { 4 const commandResult = await new Command( 5 "printenv", 6 variableName 7 ).execute(); 8 9 if (commandResult.code !== 0) {10 throw new Error(commandResult.stderr);11 }12 13 return commandResult.stdout;14}
The example is in Typescript, but can be easily transformed in standard JavaScript.
The Command
API is quite powerful, so you can read its documentation to adapt the code to your needs.
Requirements
There are another couple of things you should consider: first, you need to have installed in your frontend environment the @tauri-apps/api
package. You also need to enable your app to execute commands. Since Tauri puts a strong emphasis on the security (and rightly so!), your app is by default sandboxed. To being able to use the Command
API, you need to enable the shell.execute
API.
It should be as easy as setting tauri.allowlist.shell.execute
to true
in your tauri.json
config file.
Tauri is very nice, and I really hope it will conquer the desktops and replace Electron, since it is lighter, faster, and safer!
Questions, comments, feedback, critics, suggestions on how to improve my English? Leave a comment below, or drop me an email at [email protected].
Ciao,
R.
Comments